We are going to look at a short (and completely useless from a production standpoint) example of exposing a web service using JAX-WS.
We will create a simple method to get a Single Employee data. You can then build from there to further enhance the functionality.
We are not going to look into deploying this web service on a web server. That is for a separate post.
Lets Dive in:
We will first create a simple maven project in eclipse: ExampleWSService
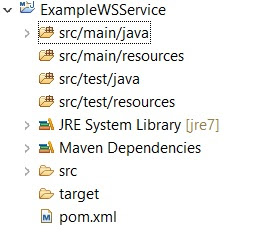
First add your maven dependency:
Create a new interface EXWSService which will serve as our SEI (service endpoint interface). This is not a a necessary step but it is always good to specify your exposed methods in an interface and then have an implementation class for the business logic.
We will create a simple method to get a Single Employee data. You can then build from there to further enhance the functionality.
We are not going to look into deploying this web service on a web server. That is for a separate post.
Lets Dive in:
We will first create a simple maven project in eclipse: ExampleWSService
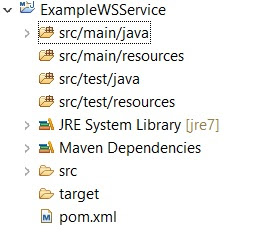
First add your maven dependency:
<dependencies>
<dependency>
<groupId>javax.xml.ws</groupId>
<artifactId>jaxws-api</artifactId>
<version>2.2.6</version>
</dependency>
</dependencies>
Create a new interface EXWSService which will serve as our SEI (service endpoint interface). This is not a a necessary step but it is always good to specify your exposed methods in an interface and then have an implementation class for the business logic.
package com.study.ws;
import javax.jws.WebMethod;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
import javax.jws.soap.SOAPBinding.Use;
import javax.xml.ws.ResponseWrapper;
import com.study.ws.domain.Employee;
@WebService(name = "EXWSService")
@SOAPBinding(style = Style.DOCUMENT, use = Use.LITERAL)
public interface EXWSService {
@WebMethod(operationName = "getEmployee", action = "getEmployee")
@ResponseWrapper(className = "com.study.ws.jaxws.GetEmployeeResponse", partName = "WSEmployee")
@WebResult(name = "employee")
public Employee getEmployee();
}
Annotations:
- @Webservice: Allows us to provide a name element and designates this interface as an end point. The name provided here will appear in the WSDL as the name of the PortType.
- @SoapBinding: This annotation allows us to specify the message style and encoding. Based on whether we use DOCUMENT or RPC style the interpretation of some of the other annotations changes. We can also select the parameterStyle, which if set to BARE means that the method parameter is the entire body of the message and there is no wrapper element.
- @WebMethod: This annotation is required on each service method that we are going to expose. We can specify the operation name and soap action.
- @ResponseWrapper: This is required if the style of SOAPBINDING is DOCUMENT. You may specify a fully qualified class name and a part name. If the style is RPC or if the parameterStyle is BARE along with style being DOCUMENT the wrapper need not be specified.
- @WebResult: You can specify the partName, name and whether the result is to be pulled from header.
We then provide an implementation class as follows:
package com.study.ws;
import javax.jws.WebService;
import com.study.ws.domain.Employee;
@WebService(endpointInterface = "com.study.ws.EXWSService", serviceName="EXWSServiceImpl", portName="EXWSServiceport")
public class EXWSServiceImpl implements EXWSService{
public Employee getEmployee() {
return new Employee(1L, "James", "Bond");
}
public EXWSServiceImpl() {
super();
}
}
Now compile your project. Go to the classes folder. In this folder (if you have used exactly the same names as in here) run the following command:
wsgen -cp . -keep -verbose com.study.ws.EXWSServiceImpl.
wsgen utility creates the request and response wrapper classes that are required with DOCUMENT style of SOAPBINDING. The @RequestWrapper and @ResponseWrapper allow you to specify the details of such generated cass. Even if these annotations are not provided default values are used and wrapper classes are generated.
You should see output as below:
Add the generated classes to your source. If you view them you will see that they just wrap your request and response data.
Next create a publisher as follows:
package com.study.ws.publisher;
import javax.xml.ws.Endpoint;
import com.study.ws.EXWSServiceImpl;
public class Publisher {
public static void main(String[] args) {
Endpoint.publish("http://localhost:8090/EXWSServiceImpl", new EXWSServiceImpl());
}
}
Run it and type the following url in your browser:
http://localhost:8090/EXWSServiceImpl
The web service is now published and you may explore the generated WSDL. To understand the WSDL and relate its segments to java annotations have a look at the following article: